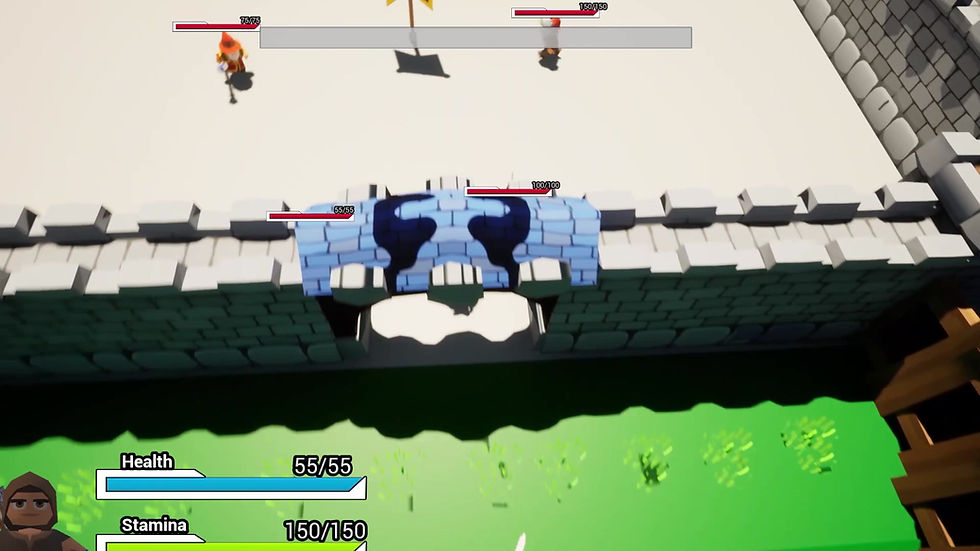
About Me
Hi I'm Benjamin Morris MSc, BSc (Hons), I am primarily a games programmer, with a varied skillset capable of C++, C#, DirectX11 and Unity and Unreal Engine Development. I also specialise in Artificial Intelligence development, being well versed in both machine learning, game AI, and applying machine learning techniques to games. I am a University Graduate with a Distinction in MSc Games Programming (by negotiated study) and a First Class Honours in BSc Computer Games Design and Programming
Portfolio
Below is some of the projects I have developed, the examples show projects within Unity, Unreal Engine, C++ and C++ using DirectX 11, some of the examples are quite long so where possible they have been edited down to a snippet of gameplay in different sections.